High End Retouching Services High End Photo Retouching exists to provide high end commercial level retouching suitable for print. We work online and internationally. We specialise in commercial, beauty, glamour, magazine & Notoriety, fashion and editorial using industry standard retouching techniques to retain and enhance texture and deliver flawless results. Retouching, photo retouching, art design, freelance photo artists, fashion artists, fashion design, fashion retouching.
Monday, July 30, 2012
Sunday, July 29, 2012
My Photo Editor
Introduction
In this article I will show you how to make a software like Photoshop except simpler, smaller and easier to use. You can load an image, change it into many color spaces (RGB, HSV, YCrCb), adjust brightness/contrast, do some filter (Noise, Blur ...), equalize histogram, view image information and so on. Image after processing can be saved with an optional quality.This small software was written using Microsoft Visual Studio 2010 and OpenCV library (version 2.4.1). To use the code, you must have some basic knowledge in image processing, know how to use MFC in Visual Studio as well as OpenCV.
Using the Code
I divide this program into 3 parts: image processing core class, image preview class and main processing.The Image processing core class contains many processing functions like adjust brightness, add noise, detect edge, rotate image and so on. All these functions have a form like this:
// Image processing function in header file
void Brightness(cv::Mat &src, cv::Mat &dst, int val)
Where
src
is source image, dst
is image after processing and val
is value for a specific function. If We name the header file the
ImgProcessing, then in the implementation file, the function above looks
like this: // ImgProcessing.cpp file
void ImgProcessing::Brightness(Mat &src, Mat &dst, int val)
{
if(src.channels() == 1)
{
for(int i = 0; i < src.rows; i++)
for(int j = 0; j < src.cols; j++)
dst.at<uchar>(i,j) = saturate_cast<uchar>(src.at<uchar>(i,j) + val);
}
if(src.channels() == 3)
{
for(int i = 0; i < src.rows; i++)
for(int j = 0; j < src.cols; j++)
for(int k = 0; k < 3; k++)
dst.at<Vec3b>(i,j)[k] = saturate_cast<uchar>(src.at<Vec3b>(i,j)[k] + val);
}
}
The image preview class is inherited from
CDialog class. This class has unity functions that allow us to change
parameters and preview what image should be like after processing. Two images above show cases of previewing (noise and distort image). In fact, there are many cases need to be previewed before any decision was made. Because each function has its own type and its own parameters, so the number of dialog preview will be nearly equal to the number of processing function! That means we must create a lot of dialog and spend more time to write the code. It is not a good choice. To avoid creating many preview dialogs and writing more code, we create just only one and give it some options. The appearance and the returned value of preview dialog will depends on what the main process told it to do.
For example, suppose we are processing the brightness of an image, when the brightness adjustment menu is clicked, the image preview dialog will appear. And the OnInitDialog function will be defined as the following:
BOOL ImgPreview::OnInitDialog()
{
// Init components
this->slider1.SetRange(0, 450);
this->slider1.SetPos(225);
this->slider2.SetRange(0, 450);
this->slider2.SetPos(225);
this->slider3.SetRange(0, 450);
this->slider3.SetPos(225);
this->slider2.EnableWindow(false);
this->slider3.EnableWindow(false);
switch(type)
{
case m_brightness:
{
this->param1.SetWindowTextA("Brightness");
}
break;
.....
}
return true;
}
When we change the position of slider on image preview dialog,
the brightness of the image must be changed and displayed on the
picture box. There are ways to display an image on controls in MFC (eg.
picture control). In this article, I use a special unity function of MFC
to force image to display. So let's create an Picture Control or
arbitrary control you like. Change the ID of control to IDC_STATIC_IMG_PREVIEW
and when the dialog is initialized, put the following code to OnInitDialog
function:BOOL ImgPreview::OnInitDialog()
{
// Init components
//....
cv::namedWindow("Image Preview", 1);
HWND hWnd = (HWND) cvGetWindowHandle("Image Preview");
HWND hParent = ::GetParent(hWnd);
::SetParent(hWnd, GetDlgItem(IDC_STATIC_IMG_PREVIEW)->m_hWnd);
::ShowWindow(hParent, SW_HIDE);
//....
return true;
}
Until now, each time we call the cv::imshow("Image Preview", image)
function,
It will force the picture control to show the image. Now, the code when
changing slider position will be as following: void ImgPreview::OnNMCustomdrawSlider1(NMHDR *pNMHDR, LRESULT *pResult)
{
LPNMCUSTOMDRAW pNMCD = reinterpret_cast<LPNMCUSTOMDRAW>(pNMHDR);
// TODO: Add your control notification handler code here
cv::Mat img_dst = img_display.clone();
switch(type)
{
case m_brightness:
{
i_param1 = slider1.GetPos() - (int)slider1.GetRangeMax()/2;
l_disp1.SetWindowTextA(ToString(i_param1));
process.Brightness(img_display, img_dst, i_param1);
cv::imshow("Image Preview", img_dst);
}
// Other cases
//...
}}
img_display
is an image which resized from source image to be smaller to display on preview dialog.If the OK button is pressed, the
is_ok
member
of ImgPreview class will be set to true to let the main process know
that everything seems alright. Otherwise, it will be set to false (This
is one way two dialogs communicate). The main process is based on MFC dialog with a menu system, buttons and other controls. Let's create a menu system like this:
And then add event handler to each menu. The
invert event handler
looks like this:void CMyPhotoEditorDlg::OnUpdateAdjustmentsInvert(CCmdUI *pCmdUI)
{
// Invert Image
process.Invert(src, src);
ImageDisplay(src);
.....
}
Where process
is an instance of ImgProcessing
class, and ImageDisplay()
is a function forced image to display in MFC control.If menu that process an image need to be previewed, menu event handlers will look this:
void CMyPhotoEditorDlg::OnUpdateAdjustmentsBrightness(CCmdUI *pCmdUI)
{
// Adjust brightness
ImgPreview dlg;
dlg.SetType(m_brightness);
dlg.src = src;
dlg.DoModal();
if(dlg.is_ok)
{
process.Brightness(src, src, dlg.i_param1);
ImageDisplay(src);
.....
}
}
Another important task is to validate menu system. If not, some
errors may occur. For example, if we try to convert image that are in
grayscale mode to hsv mode or if we try to modify image that has not
been loaded (ie empty image), program may crash. Validate menu function
is as below:void CMyPhotoEditorDlg::ValidateMenu()
{
// Validate menu
CMenu *m_file = GetMenu();
m_file->GetSubMenu(0);
CMenu *m_image = GetMenu();
m_image->GetSubMenu(1);
....
if(!is_load)
{
m_file->EnableMenuItem(ID_FILE_SAVE1, MF_DISABLED|MF_GRAYED);
m_image->EnableMenuItem(ID_MODE_GRAYSCALE, MF_DISABLED|MF_GRAYED);
....
}
else
{
m_file->EnableMenuItem(ID_FILE_SAVE1, MF_ENABLED);
m_image->EnableMenuItem(ID_MODE_GRAYSCALE, MF_ENABLED);
....
}
if(is_gray)
{
m_image->EnableMenuItem(ID_MODE_HSV, MF_DISABLED|MF_GRAYED);
m_image->EnableMenuItem(ID_MODE_YCrCb, MF_DISABLED|MF_GRAYED);
m_image->EnableMenuItem(ID_MODE_RGBCOLOR, MF_DISABLED|MF_GRAYED);
}
if(is_hsv)
{
m_image->EnableMenuItem(ID_MODE_HSV, MF_DISABLED|MF_GRAYED);
m_image->EnableMenuItem(ID_MODE_GRAYSCALE, MF_DISABLED|MF_GRAYED);
m_image->EnableMenuItem(ID_MODE_YCrCb, MF_DISABLED|MF_GRAYED);
}
....
}
The view menu contains image information, histogram and
history. If these menus are selected, they are then set to checked and
updated after each step.void CMyPhotoEditorDlg::OnUpdateAdjustmentsBrightness(CCmdUI *pCmdUI)
{
// Brightness
ImgPreview dlg;
...
dlg.DoModal();
if(dlg.is_ok)
{
....
if(is_histogram) UpdateHistogram(0);
if(is_history) history_list.AddString("Adjust Image Brightness");
}
}
Note that the explanation above is simple and just give some
main ideas, many codes accompanied with comments are easy to understand
and use.History
Version 1.0.This is the simplest program. I will comeback with an updated version.
License
This article, along with any associated source code and files, is licensed under The Code Project Open License (CPOL)
10 photo-editing tools for community managers
People are talking about the
image-powered Web—the notion that Internet users prefer the most
efficient and engaging methods of communication.
Instagram has helped fuel this trend, and now we're seeing apps like Frametastic create mini-montages to help tell visual stories in even more detail. Take, for example, the image below, which is featured on LeBron James' Facebook page.

As a result of this movement, content curators and community managers must equip themselves with a series of new tools to meet this demand. With that in mind, here are the photo-editing tools that will help community managers create the visual content their audiences crave. They’re free, although some require registration.
These sites will come in handy if you don't have access to Photoshop, which is considered the most powerful software for editing images.
The top five …
1. Pixlr Express
You will fall in love with Pixlr Express quickly. For former users of Picknik, which was acquired by Google, Pixlr Express is the perfect substitute.
It enables you to create multi-frame images, add layers, manipulate special effects, and much more. Plus, it’s incredibly easy to use. If you make one of these tools your default photo editor, make sure it’s Pixlr.

2. Wigiflip RoflBot
This tool can help you create meme-style captioned images in a matter of seconds.
Why is this important? Just take a look a the “Ted” (the film) Facebook page, and you'll get all the proof you need.
Simply head to Wigiflip RoflBot, upload your master image, and add your desired text.
Use wisely.

3. Phixr
When you arrive at Phixr.com, you might think you've landed on a simple, almost cheap looking site. But get past the initial appearance and you'll find another photo editor that will make your life easier and your communities happier.
This one also gives you the classic meme-style options, including the always-popular poster template.
4. BeFunky
BeFunky.com is another all-in-one option that enables you to make basic edits, apply artistic effects, and add graphics, shapes, speech bubbles, and text.
5. Pixisnap
If you want to create a montage or Polaroid tiles from a single picture, Pixisnap should be in your arsenal. (Keep in mind that it’s still in beta.)
Best of the rest …
6. Citryfy is a photo “enhancer” that can improve blemishes and other imperfections.
7. FotoFlexer is an advanced photo editing site/tool.
8. DrPic is a basic all-in-one editor.
9. PhotoVisi is another solid photo collage maker.
10. PinWords will instantly add text to your images.
A version of this story first appeared on AdamVincenzini.com.
Instagram has helped fuel this trend, and now we're seeing apps like Frametastic create mini-montages to help tell visual stories in even more detail. Take, for example, the image below, which is featured on LeBron James' Facebook page.
As a result of this movement, content curators and community managers must equip themselves with a series of new tools to meet this demand. With that in mind, here are the photo-editing tools that will help community managers create the visual content their audiences crave. They’re free, although some require registration.
These sites will come in handy if you don't have access to Photoshop, which is considered the most powerful software for editing images.
The top five …
1. Pixlr Express
You will fall in love with Pixlr Express quickly. For former users of Picknik, which was acquired by Google, Pixlr Express is the perfect substitute.
It enables you to create multi-frame images, add layers, manipulate special effects, and much more. Plus, it’s incredibly easy to use. If you make one of these tools your default photo editor, make sure it’s Pixlr.
2. Wigiflip RoflBot
This tool can help you create meme-style captioned images in a matter of seconds.
Why is this important? Just take a look a the “Ted” (the film) Facebook page, and you'll get all the proof you need.
Simply head to Wigiflip RoflBot, upload your master image, and add your desired text.
Use wisely.
3. Phixr
When you arrive at Phixr.com, you might think you've landed on a simple, almost cheap looking site. But get past the initial appearance and you'll find another photo editor that will make your life easier and your communities happier.
This one also gives you the classic meme-style options, including the always-popular poster template.
4. BeFunky
BeFunky.com is another all-in-one option that enables you to make basic edits, apply artistic effects, and add graphics, shapes, speech bubbles, and text.
5. Pixisnap
If you want to create a montage or Polaroid tiles from a single picture, Pixisnap should be in your arsenal. (Keep in mind that it’s still in beta.)
Best of the rest …
6. Citryfy is a photo “enhancer” that can improve blemishes and other imperfections.
7. FotoFlexer is an advanced photo editing site/tool.
8. DrPic is a basic all-in-one editor.
9. PhotoVisi is another solid photo collage maker.
10. PinWords will instantly add text to your images.
A version of this story first appeared on AdamVincenzini.com.
Nadav Kander talks about his approach to photography and portraiture
“I tend not to spend a lot of time with the sitter beforehand.”
“When I meet them it’s when they sit down or stand up in front of the camera and I let it go from there.”
“I’ve always chosen some lighting that I think is appropriate for the way that I want to see that person, but I’m often wrong.”
“Very often what I thought would be appropriate really isn’t and I rethink it. That’s when it gets interesting.”
Still Images in Great Advertising- Michael Muller
Still Images In Great Advertising, is a column where Suzanne Sease discovers great advertising images and then speaks with the photographers about it.
This week I’m talking with Michael Muller.
Suzanne: Do you think these images were the reason you were hired for this poster?
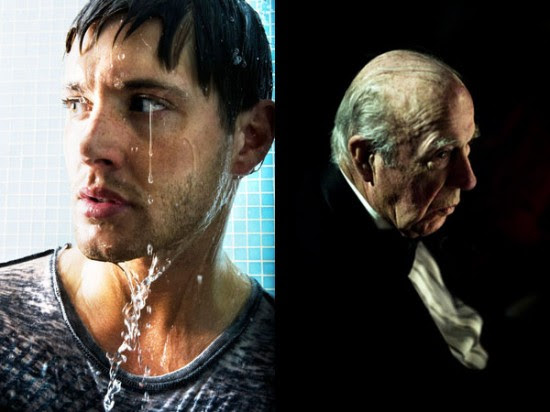
Michael: No those photos were taken years ago and when I shoot posters these days it’s due primarily to my relationships with the studios or a particular actor or director. At this point in my career they hire me for the work I do and the style I bring, not to a particular image that is right for a particular poster. They will use those types of images for mood boards or ref images but in the case of battleship it was not the case. In fact the water dripping from the actors came up on the set of the shoot, NOT in the design portion! It was actually kind of funny because a few of the big wigs from the studio etc didn’t think Rhianna would want to get all wet and she actually turned out to be the one who wanted to do it most and pushed for it on the shoot! We had to wait until the end of the shoot to do that shot or they would have had to go back to hair and makeup for hours to get made back up. As for the lighting, I have a wide range of lighting techniques in my arsenal and depending on the film and mood I want to set for a project determines the lighting I will use. On most shoots I do 4-6 different lighting set ups on each job which gives them a wide variety of looks to work with for different uses.
Suzanne: This one poster is very different than the others for this movie but I think more artistic and successful. Did you talk them into this piece?
Yes there is a certain “talking into” that takes place when your adding water or fire to a shot. It isn’t really talking into but more getting them to trust the process and that it will most likely work for the project. There are occasions were it does not work but without trying one never knows so I always push to try shots like this. I also liked the idea of water dripping down the face since the film centered around the ocean and how do you say that without shoving it down the viewers face? I like to do things in subtle ways as much as possible, and water is such an amazing substance to work with no matter what the use.
Suzanne: I love the way you push your work. If there is something you are intrigued by you put it out there. i.e. Sharks and underwater, eagle study, under water study of materials and body to the motorcycle riders. What advice would you give to photographers to show their personal work that still reflects the work they want to be hired for?
It is interesting because take movie posters for example, I wanted to shoot these 6 years ago or something and tried for years asking peoples advice on how to break into them and got so many suggestions such as “shoot on white that’s what they want to see” or “ask your actor friends” as well as many others and none of those worked. What no one suggested was to “Go down to Hollywood Blvd and spend 3 months documenting those freaks that hang out in front of the Chinese Theatre in costumes and pose with tourist for 5$ and do a Gallery show on them. Get Batman smoking crack in a back alley and a storm trooper having a smoke break etc. Then get a big actor to buy a print and hang it on his wall and when the head of marketing from one of the studios comes over to go over a film he’s doing he will see it and hire you on the spot for the biggest movie of the year for that studio” do that Michael and you will get into Movie posters!! That is what did happen and that was just following my gut/heart and shooting something I thought was cool.
AS for sharks etc, I am passionate about those and as a photographer I shoot anything I am passionate about. If you fall into the trap of only picking up your camera for work or a paycheck your going to find yourself screwed and or compromising as an artist. Those things are what keep my fire going, keeps me smiling and I LOVE the challenge especially of shooting things in different ways then have been done before. That is what led to the patent I have on the most powerful underwater strobe lights in the World! Yes A patent, made something new that didn’t exist before that is allowing me to shoot things in ways NEVER done before! it’s truly inspiring to me and makes me long to shoot more and more even after 27 years of doing this!!!
Suzanne: Please tell me more about your Kids Clicking Kids mission?
It is where we bring photography into Hospitals with the Art of Elysium and watch kids enjoy and use the medium. Watching smiles come on these kids faces that are in so much pain and get a momentary escape is priceless. There are so many people and animals out there that need help and if everyone did something no matter how small this World would be such a better place. I think that most people just assume someone else is going to do it or they are “too Busy” keeping up with the Jones and chasing the all mighty dollar and don’t realize the real pay off is in giving. The most powerful result from a photo I have ever taken comes from not a photo but giving that experience away to someone else. You get so much more back from that than any billboard that only feeds the ego or the pocket book.
Suzanne: I really appreciate that you don’t have a bio on your website but more about the charities that are very important to you. You really seem to be involved in multiple of charities. That alone would want me to hire you. Tell me more about your philosophy of giving back.
I work with many charities and always give prints when called upon for ones that I don’t work with. I was just made a Global Advocate of the United Nations which I work with in a very active way. I just went with the UN to Africa to highlight Malaria. My images help tell the stories of these people that have pretty much been put in a corner of the World and forgotten about. They feel invisible and by taking their picture they no longer are invisible but there is proof they are HERE and they EXIST!! I work with many Ocean Org such as Sea Sheperds and recently have teamed up with Philippe Costeau and his Earth Eco org that helps educate children about our planet and oceans!
http://www.variety.com/article/VR1118054204
http://www.nothingbutnets.net/
http://www.earthecho.org/
http://www.seashepherd.org/events/sea-no-evil-art-show-august-29-2009.html
http://hellogiggles.com/item-of-the-day-368
Note: Content for Still Images In Great Advertising is found. Submissions are not accepted.
Michael is represented by Stockland Martel.
APE contributor Suzanne Sease currently works as a consultant for photographers and illustrators around the world. She has been involved in the photography and illustration industry since the mid 80s, after founding the art buying department at The Martin Agency then working for Kaplan-Thaler, Capital One, Best Buy and numerous smaller agencies and companies..
This week I’m talking with Michael Muller.
Suzanne: Do you think these images were the reason you were hired for this poster?
Michael: No those photos were taken years ago and when I shoot posters these days it’s due primarily to my relationships with the studios or a particular actor or director. At this point in my career they hire me for the work I do and the style I bring, not to a particular image that is right for a particular poster. They will use those types of images for mood boards or ref images but in the case of battleship it was not the case. In fact the water dripping from the actors came up on the set of the shoot, NOT in the design portion! It was actually kind of funny because a few of the big wigs from the studio etc didn’t think Rhianna would want to get all wet and she actually turned out to be the one who wanted to do it most and pushed for it on the shoot! We had to wait until the end of the shoot to do that shot or they would have had to go back to hair and makeup for hours to get made back up. As for the lighting, I have a wide range of lighting techniques in my arsenal and depending on the film and mood I want to set for a project determines the lighting I will use. On most shoots I do 4-6 different lighting set ups on each job which gives them a wide variety of looks to work with for different uses.
Suzanne: This one poster is very different than the others for this movie but I think more artistic and successful. Did you talk them into this piece?
Yes there is a certain “talking into” that takes place when your adding water or fire to a shot. It isn’t really talking into but more getting them to trust the process and that it will most likely work for the project. There are occasions were it does not work but without trying one never knows so I always push to try shots like this. I also liked the idea of water dripping down the face since the film centered around the ocean and how do you say that without shoving it down the viewers face? I like to do things in subtle ways as much as possible, and water is such an amazing substance to work with no matter what the use.
Suzanne: I love the way you push your work. If there is something you are intrigued by you put it out there. i.e. Sharks and underwater, eagle study, under water study of materials and body to the motorcycle riders. What advice would you give to photographers to show their personal work that still reflects the work they want to be hired for?
It is interesting because take movie posters for example, I wanted to shoot these 6 years ago or something and tried for years asking peoples advice on how to break into them and got so many suggestions such as “shoot on white that’s what they want to see” or “ask your actor friends” as well as many others and none of those worked. What no one suggested was to “Go down to Hollywood Blvd and spend 3 months documenting those freaks that hang out in front of the Chinese Theatre in costumes and pose with tourist for 5$ and do a Gallery show on them. Get Batman smoking crack in a back alley and a storm trooper having a smoke break etc. Then get a big actor to buy a print and hang it on his wall and when the head of marketing from one of the studios comes over to go over a film he’s doing he will see it and hire you on the spot for the biggest movie of the year for that studio” do that Michael and you will get into Movie posters!! That is what did happen and that was just following my gut/heart and shooting something I thought was cool.
AS for sharks etc, I am passionate about those and as a photographer I shoot anything I am passionate about. If you fall into the trap of only picking up your camera for work or a paycheck your going to find yourself screwed and or compromising as an artist. Those things are what keep my fire going, keeps me smiling and I LOVE the challenge especially of shooting things in different ways then have been done before. That is what led to the patent I have on the most powerful underwater strobe lights in the World! Yes A patent, made something new that didn’t exist before that is allowing me to shoot things in ways NEVER done before! it’s truly inspiring to me and makes me long to shoot more and more even after 27 years of doing this!!!
Suzanne: Please tell me more about your Kids Clicking Kids mission?
It is where we bring photography into Hospitals with the Art of Elysium and watch kids enjoy and use the medium. Watching smiles come on these kids faces that are in so much pain and get a momentary escape is priceless. There are so many people and animals out there that need help and if everyone did something no matter how small this World would be such a better place. I think that most people just assume someone else is going to do it or they are “too Busy” keeping up with the Jones and chasing the all mighty dollar and don’t realize the real pay off is in giving. The most powerful result from a photo I have ever taken comes from not a photo but giving that experience away to someone else. You get so much more back from that than any billboard that only feeds the ego or the pocket book.
Suzanne: I really appreciate that you don’t have a bio on your website but more about the charities that are very important to you. You really seem to be involved in multiple of charities. That alone would want me to hire you. Tell me more about your philosophy of giving back.
I work with many charities and always give prints when called upon for ones that I don’t work with. I was just made a Global Advocate of the United Nations which I work with in a very active way. I just went with the UN to Africa to highlight Malaria. My images help tell the stories of these people that have pretty much been put in a corner of the World and forgotten about. They feel invisible and by taking their picture they no longer are invisible but there is proof they are HERE and they EXIST!! I work with many Ocean Org such as Sea Sheperds and recently have teamed up with Philippe Costeau and his Earth Eco org that helps educate children about our planet and oceans!
http://www.variety.com/article/VR1118054204
http://www.nothingbutnets.net/
http://www.earthecho.org/
http://www.seashepherd.org/events/sea-no-evil-art-show-august-29-2009.html
http://hellogiggles.com/item-of-the-day-368
Note: Content for Still Images In Great Advertising is found. Submissions are not accepted.
Michael is represented by Stockland Martel.
APE contributor Suzanne Sease currently works as a consultant for photographers and illustrators around the world. She has been involved in the photography and illustration industry since the mid 80s, after founding the art buying department at The Martin Agency then working for Kaplan-Thaler, Capital One, Best Buy and numerous smaller agencies and companies..
This Week In Photography Books – Lucas Foglia
By coincidence, I was in New York the day after Lehman Brothers crashed, back in 2008. Fear was in the air. Not a nice smell.
I sat in a Hungarian pastry shop near Columbia University, downing cup after cup of diner-style coffee, chatting with a friend. His name is Ivan, and he’s the only person I’ve ever met who introduced himself as a Mexican Marxist Yankee Fan. Top that.
He was my professor in graduate school, an expert in the Globalized Economy. We stayed in touch after I left school, but this was our first meeting in 4 years. Each sip of the weak, caffeinated beverage sped the pace of our speech. Spittle flew, hair was tossed, eyes ablaze.
In the end, we agreed that the Global Economic System would not collapse. The crisis, in it’s purest form, was not yet 24 full hours old. Still, we thought it through, and both believed that there was too much money in the system, too much at stake, for Chaos to Reign. Whosoever had any money left at all, be it the Chinese, the Oil Kingdoms, the Russians…little matter. Money would, in the end, protect itself.
I clung to that belief as the markets fell. It will get better. It will get better. Back home, my in-laws would make off-handed comments, like, “Well, at least we have lots of water, and we can always grow our own food on the farm.” Or other times, someone would say, “At least Tim (my brother-in-law) knows how to hunt. A big elk can last a long time.” Not. Very. Re-assuring.
By now, we all know it never came to that. The system defended itself, though, of course, many still suffer. Still, the milk trucks run, McDonalds cranks out it’s faux-burger-patties, and now we have Facebook. It’s hard to channel the depths of that early fear, but I remember it’s smell.
There are those, though, who need not fear a system crash. They eschew the system, and re-create the old ways. Living off the land, beards aflowing. We have lots of folks like that in Taos, and we call them hippies. Most of them live on the Mesa, where the water flows 600 feet beneath them. Good luck drilling through that.
But that’s all I know of such communities: local gossip and hearsay. Not much to go on. And the little I’ve seen makes me root for the system to chug along a bit longer. I don’t think I’d like the taste of bony rabbit, but you never know.
That said, I was fascinated to get a glimpse inside the lifestyle, courtesy of Lucas Foglia’s new book “A Natural Order,” published by Nazraeli Press. It’s a straight-forward, very well produced volume that settles down into a group of off-the-grid communities in the Southeast of the United States. Fascinating stuff.
The first test that I give a book, when I pluck it from my stack, is, do I want to see more? Is there a need to turn the page? Do the pictures build to something, or can I get a good sense of the thing from the first 10 pages? You’d be surprised how many books, by great artists, are not designed to hold attention. Simply to show off the plates. (Just this morning, I set down a book by Daido Moriyama for that very reason. A big name artist does not guarantee a great book.)
When I picked this one up, though, I was captured, and transported. Ironically, I’d seen some of these images before on the Internet, and was unimpressed. But a book is a thing, with a built-in structure. Not a few illuminated pixels on a screen. And in book form, this work shines.
I’d guess that the artist was using a large-format camera, given the sharpness and clarity of the photographs. But the angles and setting, deep in the woods, would have made that a difficult proposition. Either way, kudos to the image quality.
The photo on the cover shows a young red-headed lad, in the woods, holding up a big cast iron skillet filled with mystery meat. The title, given later, confirms that it’s possum. Yummy, yummy possum. (I think I’ll keep my refrigerator, thanks.)
After the title page, the artist delivers a short statement about his upbringing. Apparently, his family lived off the land, not far from NYC. But they didn’t take it as far as the subjects of the book. So Mr. Foglia, curious to see his how far the lifestyle could be pushed, set out to discover the answers for himself. That is how it’s done.
The pictures are well-composed, and slowly build together the details that matter. Animal skins covering windows, teepees popped up alongside pretty lakes. Guns, and bows and arrows, and chainsaws and women with underarm hair. Water serves to bathe, but also as a mirror for a man checking out his new haircut. An oxplow is pulled by a Toyota pickup truck, a boy drinks raw goat milk from the teat, and a poisoned dead bear rots on the ground. (We also see a token boob shot. Remember, Boobs Sell Books.℠ To be fair, it’s balanced with two penis shots, one belonging to the perfect cross between Chris Robinson and Jesus.)
It’s a seamless vision, clad in cloth, of some people who don’t conform to the standards of the majority. Will you be curious to see this book? I don’t know. Will you?
Bottom Line: Happy Hippies, one possum at a time
To purchase “A Natural Order” visit Photo-Eye
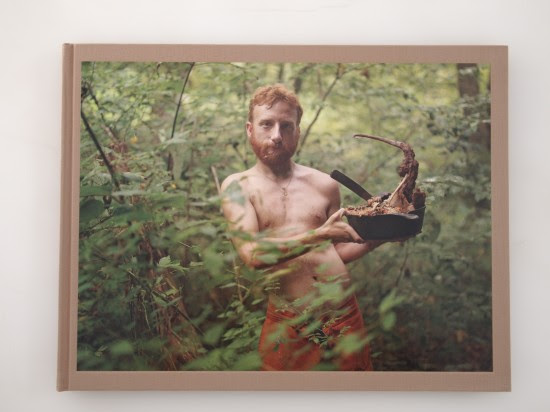
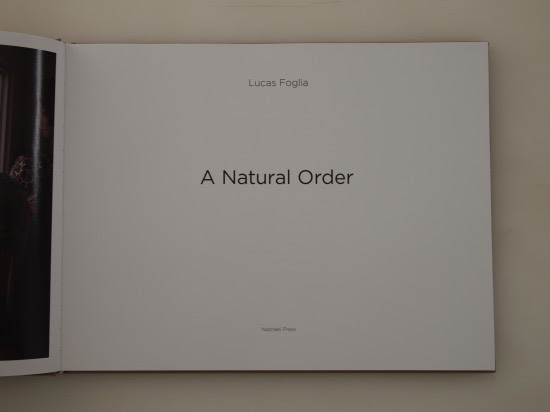
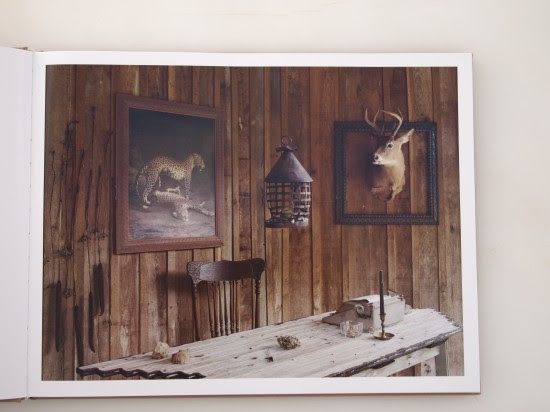
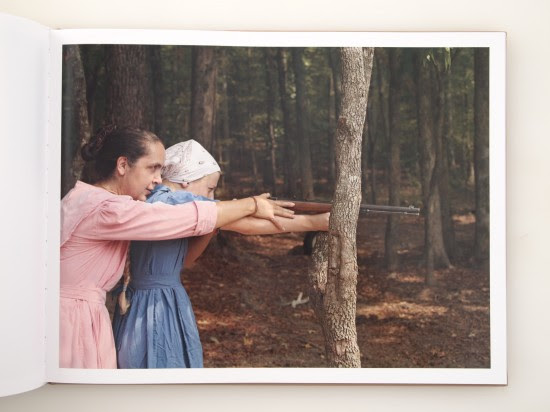
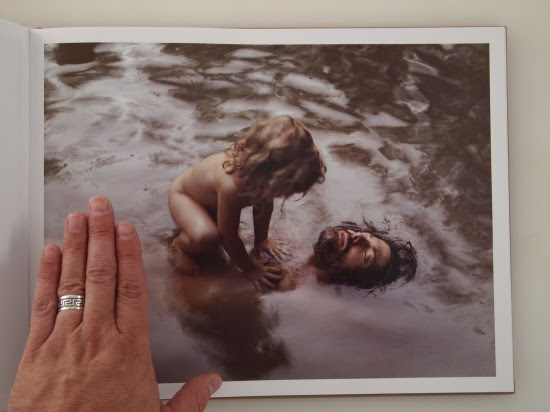
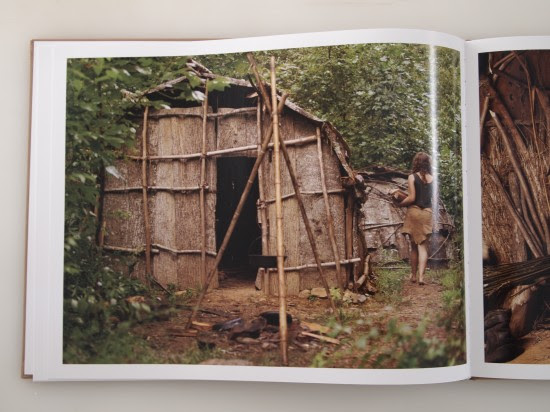
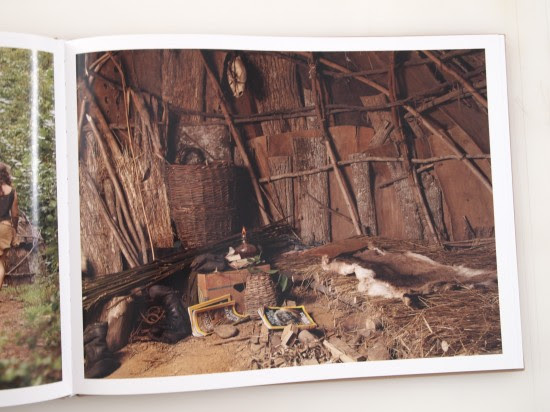
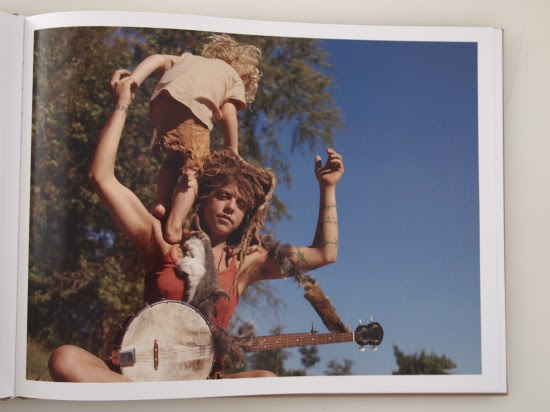
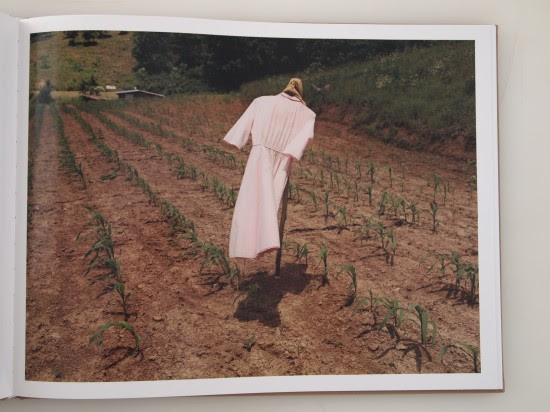
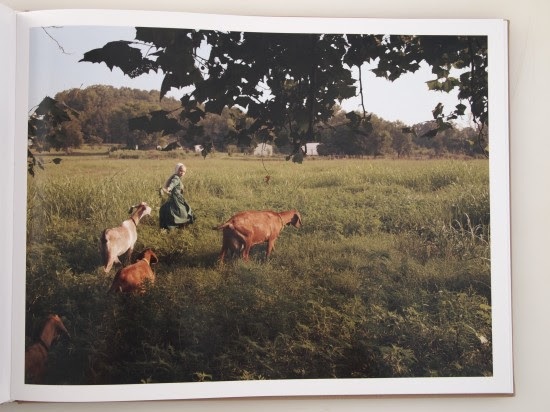
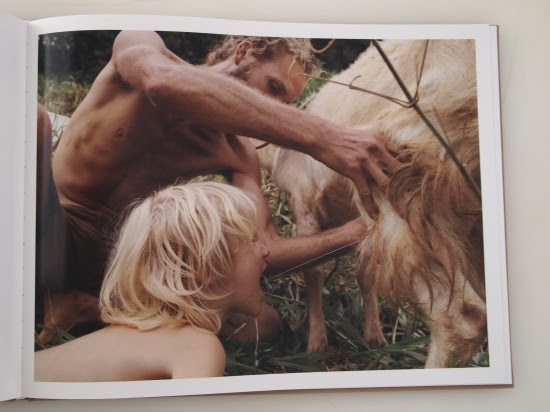
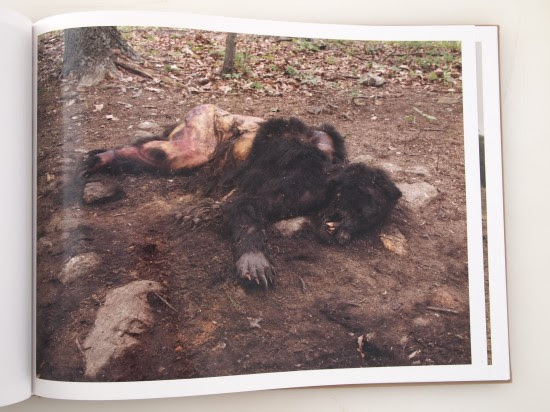

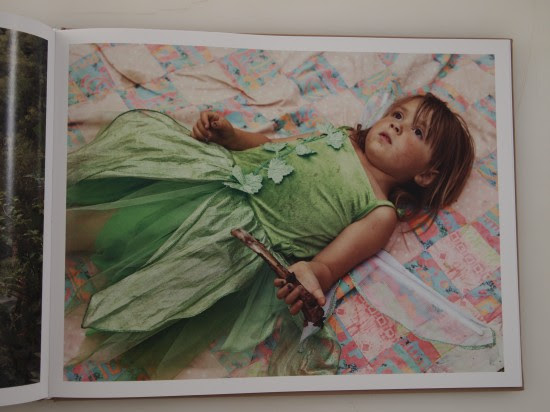
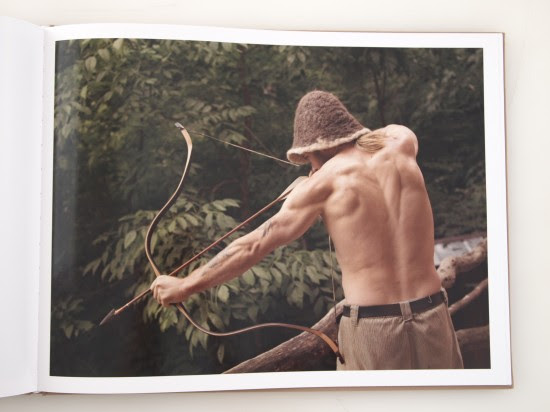
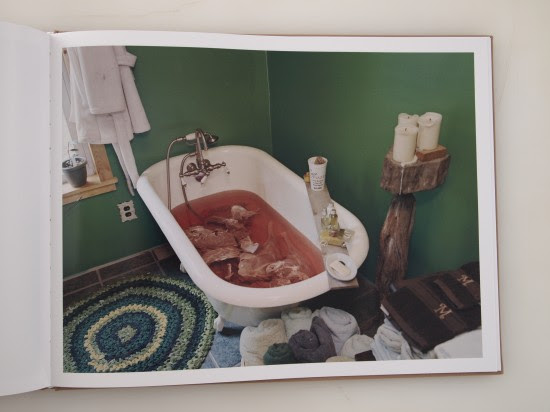
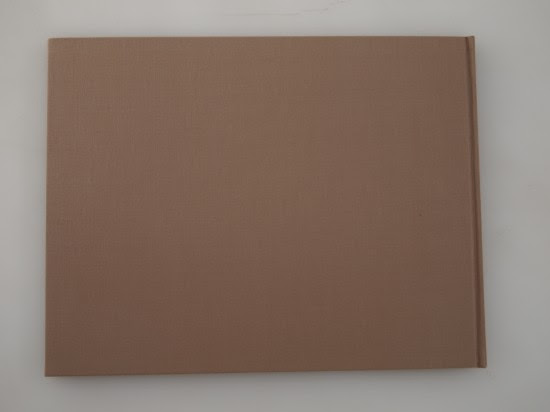
Full Disclosure: Books are provided by Photo-Eye in exchange for links back for purchase.
Books are found in the bookstore and submissions are not accepted.
I sat in a Hungarian pastry shop near Columbia University, downing cup after cup of diner-style coffee, chatting with a friend. His name is Ivan, and he’s the only person I’ve ever met who introduced himself as a Mexican Marxist Yankee Fan. Top that.
He was my professor in graduate school, an expert in the Globalized Economy. We stayed in touch after I left school, but this was our first meeting in 4 years. Each sip of the weak, caffeinated beverage sped the pace of our speech. Spittle flew, hair was tossed, eyes ablaze.
In the end, we agreed that the Global Economic System would not collapse. The crisis, in it’s purest form, was not yet 24 full hours old. Still, we thought it through, and both believed that there was too much money in the system, too much at stake, for Chaos to Reign. Whosoever had any money left at all, be it the Chinese, the Oil Kingdoms, the Russians…little matter. Money would, in the end, protect itself.
I clung to that belief as the markets fell. It will get better. It will get better. Back home, my in-laws would make off-handed comments, like, “Well, at least we have lots of water, and we can always grow our own food on the farm.” Or other times, someone would say, “At least Tim (my brother-in-law) knows how to hunt. A big elk can last a long time.” Not. Very. Re-assuring.
By now, we all know it never came to that. The system defended itself, though, of course, many still suffer. Still, the milk trucks run, McDonalds cranks out it’s faux-burger-patties, and now we have Facebook. It’s hard to channel the depths of that early fear, but I remember it’s smell.
There are those, though, who need not fear a system crash. They eschew the system, and re-create the old ways. Living off the land, beards aflowing. We have lots of folks like that in Taos, and we call them hippies. Most of them live on the Mesa, where the water flows 600 feet beneath them. Good luck drilling through that.
But that’s all I know of such communities: local gossip and hearsay. Not much to go on. And the little I’ve seen makes me root for the system to chug along a bit longer. I don’t think I’d like the taste of bony rabbit, but you never know.
That said, I was fascinated to get a glimpse inside the lifestyle, courtesy of Lucas Foglia’s new book “A Natural Order,” published by Nazraeli Press. It’s a straight-forward, very well produced volume that settles down into a group of off-the-grid communities in the Southeast of the United States. Fascinating stuff.
The first test that I give a book, when I pluck it from my stack, is, do I want to see more? Is there a need to turn the page? Do the pictures build to something, or can I get a good sense of the thing from the first 10 pages? You’d be surprised how many books, by great artists, are not designed to hold attention. Simply to show off the plates. (Just this morning, I set down a book by Daido Moriyama for that very reason. A big name artist does not guarantee a great book.)
When I picked this one up, though, I was captured, and transported. Ironically, I’d seen some of these images before on the Internet, and was unimpressed. But a book is a thing, with a built-in structure. Not a few illuminated pixels on a screen. And in book form, this work shines.
I’d guess that the artist was using a large-format camera, given the sharpness and clarity of the photographs. But the angles and setting, deep in the woods, would have made that a difficult proposition. Either way, kudos to the image quality.
The photo on the cover shows a young red-headed lad, in the woods, holding up a big cast iron skillet filled with mystery meat. The title, given later, confirms that it’s possum. Yummy, yummy possum. (I think I’ll keep my refrigerator, thanks.)
After the title page, the artist delivers a short statement about his upbringing. Apparently, his family lived off the land, not far from NYC. But they didn’t take it as far as the subjects of the book. So Mr. Foglia, curious to see his how far the lifestyle could be pushed, set out to discover the answers for himself. That is how it’s done.
The pictures are well-composed, and slowly build together the details that matter. Animal skins covering windows, teepees popped up alongside pretty lakes. Guns, and bows and arrows, and chainsaws and women with underarm hair. Water serves to bathe, but also as a mirror for a man checking out his new haircut. An oxplow is pulled by a Toyota pickup truck, a boy drinks raw goat milk from the teat, and a poisoned dead bear rots on the ground. (We also see a token boob shot. Remember, Boobs Sell Books.℠ To be fair, it’s balanced with two penis shots, one belonging to the perfect cross between Chris Robinson and Jesus.)
It’s a seamless vision, clad in cloth, of some people who don’t conform to the standards of the majority. Will you be curious to see this book? I don’t know. Will you?
Bottom Line: Happy Hippies, one possum at a time
To purchase “A Natural Order” visit Photo-Eye
Full Disclosure: Books are provided by Photo-Eye in exchange for links back for purchase.
Books are found in the bookstore and submissions are not accepted.
Forum says it will publish gay marriage notices
The newspaper was criticized locally and online for refusing to publish the marriage notice of a lesbian couple who plan to marry in New York, where gay marriage is legally recognized. By: Herald Staff Report, Grand Forks Herald
Allison Johnson, left, and Kelsey Smith meet Tuesday, July 25,
2012, with Forum Editor Matt Von Pinnon at The Forum in Fargo N.D. They
plan to marry in Aug. 1 in New York. Michael Vosburg / Forum Photo
Editor
“Starting today, The Forum will accept for publication the announcements of gay marriages, engagements and anniversaries if the marriage takes place in a state or country where it’s legally recognized,” Editor Matt Von Pinnon wrote in his column.
The newspaper won’t take sides in the debate, but its mission is to provide information to the public, which includes marriages, he wrote.
The couple that was turned away — Kelsey Smith, 27, Fargo, and Allison Johnson, 31, Austin, Texas — plans to marry in New York, where same-sex marriages are legal.
Previously, Von Pinnon has said The Forum used as “guiding principles” laws in Minnesota and North Dakota, which do not recognize gay marriages.
More than 600 people contacted The Forum, some agreeing with its former policy of not an-nouncing gay marriages and some disagreeing, according to the column.
Dividing issue
“It’s safe to say that people in our region are equally divided over the issue of gay marriage, a debate that goes well beyond the question of whether this newspaper should publish such announcements,” Von Pinnon wrote.
He wrote that “a good newspaper mirrors its community,” but, in the end, “this policy review came down to one thing: We inform the public, plain and simple. Except for what’s found on the Opinion page, we don’t choose sides. We report on many, many things that we neither endorse nor condemn. That’s the nature of news. Some people would like us to deny that gay marriage is legally recognized in several states and countries. To not recognize that fact is to deny or distort the truth, something we’re not willing to do.”
Among newspapers in major cities in North Dakota, the Herald and the Bismarck Tribune also print gay marriage announcements. The editor of the Minot Daily News has said his newspaper would look to state law for guidance.
The Herald, which, like The Forum, is owned by Forum Communications, first printed an announcement of a “commitment ceremony” in 2003.
The Forum will not go as far.
“We will not publish announcements for non-legally recognized marriages, such as civil unions and commitment ceremonies,” Von Pinnon wrote.
“We know that for some people this policy change doesn’t go far enough,” he wrote. “For others, it goes too far. We think it’s a reasonable announcement policy for the vast majority our community”
The Fine Line between Retouching and Distortion
As I’ve mentioned before, retouching is nothing new in photography. Cameras capture something close to what our eyes see, but sometimes not exactly quite right and we work in post to add that “human” quality. Or sometimes, we are trying to make up for user error; perhaps our exposure was off (too light or too dark) or maybe our white balance (the photo is too “cool” or too “warm”). Other times, we retouch because it makes the subject more…palatable. Let me explain.
On many occasions, I’ve been asked by my friends to take headshots for them. Some are actors looking to break into New York City’s theatre world while others are simply professionals in need of a photo for business purposes. None of these friends have explicitly asked me to retouch their photos, but I have gone ahead and added some “digital makeup” to augment their photos. I believe a headshot should show the person in their best possible light. If I can do something to help that, I will.
That said, I think there’s a very clear line here. People should still look like themselves. Anything I could potentially do with makeup — mask a pimple, smooth and even out skin tone, reduce shine — I’m comfortable doing in retouching. Most of my friends who have received my retouched photos do not realize they’ve been retouched. The idea here is to be subtle lest people look at their photos and think, “Gee, do I really look like that?!”
To drive home this point, I have a couple examples of helpful retouching and one example that, to me, is clearly distortion.
Example No. 1 of Retouching - Slight Retouching
The above is a perfect example of very slight retouching. Personally, I didn’t think this example needed any retouching (our Anthony has some pretty amazing skin!). But I did a very slight adjustment of the skin under Anthony’s left eye (right side of the frame) to straighten out a wrinkle. Otherwise, the photos are pretty much identical.
Example No. 2 of Retouching - More Retouched
The above is a photo I took for one of my actor friends. While I tend to glance over the imperfections of my friends (because I love them dearly!), the camera is not always as generous. In post-processing this photo, I noticed that he had a few small blemishes so I faded those away. I also removed shine and smoothed out his complexion using the post-processing tools available to me since I didn’t have a makeup artist on hand that day to prep his skin for the camera. Finally, I sharpened the focus around his eyes and brightened up the photo considerably to highlight his features. Though this sounds like a lot, the results are fairly subtle in my opinion.
Example No. 3 of Retouching - Overly Retouched
Finally, the above is a photo of myself. I took a self-portrait a couple of years ago that I felt turned out pretty well and accurately represents me (though my highlights have faded, I still wear my hair this way and still have the same glasses — I guess it might be time for a new pair!). The photo above at left is what I currently use as my headshot. The retouched version at right is, quite frankly, an abomination. It’s starting to look like a different girl, not me.
The first big thing I changed here are my eyes. If you’ve met me, you know that I have very dark eyes; and if you’ve seen my baby pictures, that’s something about me that has always been true. In this retouched photo, I lightened my eyes considerably so that it looks like I might have hazel eyes (which I don’t). And speaking of eyes, I also altered the color of my glasses. Not only is this untrue but glasses say something about a person. I’ve had pink, red, purple, brown glasses, but never green. I think that says something about my preferences and the kind of person I am (I’m bold, but not quite so bold as to wear green glasses most days of the week!).
In addition, I’ve also gone ahead and removed all the blemishes on my forehead (trust me, those are still on my current forehead!) and chin and smoothed out the skin tone. That would have been okay but then I decided to take it a step further and darken my skin tone — a digital base tan, if you will. While I tan fairly well and tend to take on color during the summer, most of the year I’m pretty pasty and the photo on the left is pretty accurate to what my skin normally looks like. I then did the inverse for my hair. Instead of darkening, I lightened. While I’ve experimented with hair color (there was a time Anthony called me “fire head” due to my hair matching the color of a lit match!), I can’t say it has ever looked that shade of brown. The sun might brighten somewhat, but again, my hair is fairly dark brown and aside from highlights every so often (when I get bored and forget how annoying those are to grow out), I really don’t do much to my hair’s natural color.
As a result of all this retouching, the picture of me on the right really doesn’t look much like me at all. It’s a distortion. She could be my alter-ego; a sort of Bizarro Jen from another dimension. That said, I think it’s sad how often people measure themselves up against distortions as much of what we see in beauty and fashion magazines is artificial in this way. Much like it would be unrealistic for me to compare my hair to that of a Barbie doll, it is unrealistic for me to compare my skin to that of a celebrity I see in a Lancome ad. More often than not, she’s not born with and it’s probably not Maybelline — it’s Photoshop.
RETOUCHING A QUARTER POUNDER
We’ve posted a number of examples that make it clear just how re-touched the images ofpeople we see in magazines and ads are. Of course, everything else in those images is photoshopped too, leading to those “hmmm, this doesn’t look quite like it does on the box” moments.
McDonald’s Canada released a video showing a photoshoot for a hamburger. It reveals the techniques that are used to get that luscious, huge, fresh look that so tempts us in food ads. I think it’s great to add to the examples of retouching people to spark discussion on our relationship to the manipulated images around us and the effects of different types of retouched images.
Thanks to Dmitriy T.C. for the tip!
onOne Software Perfect Portrait: Retouching With An “Easy” Button
Judging by the popularity of facial retouching software, there
seem to be a lot of people out there who want to make their subjects
look like they just arrived off a private jet from Monte Carlo. And they
want to do it fast, and not get bogged down with little technicalities
like learning how to use Photoshop. So, is it possible to just press a
button and instantly have a complexion that looks like J.Lo after an
hour in the makeup chair? Well, that’s what we’re here to find out, so
let’s take a look.
What Perfect Portrait claims to do is to quickly and easily retouch not just one but multiple faces in an image by automatically recognizing not only the faces in the image but the eyes and lips also so they get their own treatment. Of course, if that isn’t to your liking, you can dig deeper into the tools and make manual adjustments. Default settings are those that the manufacturer has determined will work in most situations but you can set your own presets. For example, you may want to go a little heavier on teenage girls than executive men in their 40s, so you can save these settings. There are several presets for Female, Male, Children, and Group already included.
In the suite, you open the program and it goes to the Layers section by default. Then you open an image and use whichever program you’d like. If you opt to go with just Perfect Portrait without the suite, when you open the program you are first asked to open an image and then it opens the image in the program. No big deal, you say? Well, maybe not on the beginning part but each time you are done working on an image, after you save it, the program closes and you have to go through the process again! I found that to be both annoying and slower than just opening images in an already open program.
Let’s go through a sample workflow situation here on a single image using Adobe Lightroom. The program suggests you do any major color corrections and tonal adjustments first in your program of choice, a little strange because then the program will automatically make color adjustments. Maybe the emphasis here is on major. I found the easiest way was to import the file in Lightroom, do any quick adjustments there, then go to Perfect Portrait from the File/Plugin Extras menu. The program will automatically go to work on your image and if you like what you see, you’re done! If you think it should be fine-tuned, choose one of the many presets included under the proper category. For example, if you’re retouching a man’s portrait, there are 12 presets included that range from Default, Elderly, Twilight, Warm, and more. The default menu shows up as a thumbnail bar across the bottom of the program and you can click on and apply whichever one you feel suits the image best. Once you’ve got the basics done, you can either quit there or use the tools to do more work.
One thing I found very useful was the ability to see just what the program was masking out using the Overlay view. Once again, if you are happy with the area the program selected, then leave it alone. If not, you can paint in and out using the Brush tool to have the exact area you want.
When you are done refining your image with the tools, then you advance to the slider menus on the right side of the interface. Also there you’ll find a Navigator and hot keys to select the face magnification, a Layer palette for each face, and sliders for fine-tuning skin color, shine, and other features.
I found the Face Size feature perhaps incorrectly named. Under that menu it lists Small, Medium, Large, and Extra Large, like you’re buying a man’s shirt. When I first saw it, I thought maybe it changed the shape of the face. Not so, as already noted. What it does is define how tightly or loosely you’d like the automatic face detection feature to work. Small will crop in really closely while Extra Large will stray further into the neck, hair, and other features. While I think it’s a good idea, especially if you’re trying to batch process images and don’t want to look at everyone, maybe either a number system or words like Tight or Loose would make more sense.
Let’s take a look now at how Perfect Portrait handles multiple faces in an image. When you open an image with multiple faces, the software automatically detects each face and places it on its own layer. If for some reason it missed a face, usually because it was not facing the camera, you can add the face manually. I did try to see how it would do with an image with 11 faces in a full-length pose. No faces were selected, so there are limits! But I then pulled up an image with three faces and it worked just fine. You can work on each face on its own layer independently.
You also have options when saving the image. You can just hit the Save button and your file will be saved as a PSD layer, your original file on the bottom layer and the retouched file on top. This is in keeping with nondestructive editing so your untouched original can always be accessed. You also have the option of using Export from the File menu to save as a TIFF or flattened JPEG file.
You see, I can open Lightroom, import a folder of images, export them to Perfect Portrait, choose the options I’d like, and press Export once again. VoilĂ ! All images are pretouched. I put four JPEGs in a folder to test and it went through them flawlessly. It did take a little while even with JPEGs so if you have lots of images to do you may want to hit the Export button and then go have your Frosted Mini-Wheats! And speaking of time, I have a rather new computer I used to test this. It has 4GB of RAM and a quad processor so take the minimum specs required pretty seriously, as this program does take some processing power.
Like most current software companies, onOne has extensive support online. They have a Knowledge Base that allows you to search for answers to common questions and e-mail support. They also have a feature I found most useful, onOne University, which has many tutorial videos. Because of the newness of the program, there are only a few on Perfect Portrait, but they do a good job of explaining the basics and will get you up and running quickly.
Note: It is recommended that you use an Intel Core 2 Duo, Xeon, or better processor. In addition, 8GB of RAM is recommended, while 4GB of RAM is the minimum you should use.
Perfect Portrait sells for $99.95, with an upgrade price of $69.95. Perfect Photo Suite 6 sells for $299.95, with an upgrade price of $149.95. For more information, visit onOne Software’s website at: www.ononesoftware.com.
Courtesy of onOne Software
First, let’s take a look at what we have. Perfect Portrait is one of
six products that combined give you onOne Software’s Perfect Photo Suite
6. You can buy the whole bundle or you can buy just the individual
products that you like. And while I’m not going to address the other
products here, let me just add kudos to onOne for using the same
interface for each product, something you’d think would be automatic
with software suites but sadly is not.What Perfect Portrait claims to do is to quickly and easily retouch not just one but multiple faces in an image by automatically recognizing not only the faces in the image but the eyes and lips also so they get their own treatment. Of course, if that isn’t to your liking, you can dig deeper into the tools and make manual adjustments. Default settings are those that the manufacturer has determined will work in most situations but you can set your own presets. For example, you may want to go a little heavier on teenage girls than executive men in their 40s, so you can save these settings. There are several presets for Female, Male, Children, and Group already included.
Here’s the very simple and organized
interface you see when you open an image in Perfect Portrait. Tools are
on the left, sliders on the right. The brackets on the image show the
face selected, the hot buttons under the Navigator allow you to quickly
move in tight on the face and if a face was not detected you can
manually select it. The floating palette contains fine-tuning options
that change with the tool you’ve selected. Model: Gabrielle Valesco.
All Photos © Steve Bedell
All Photos © Steve Bedell
Changing the view from Current to Overlay
allows you to see the mask that was automatically applied and to make
changes to it if desired.
The view changes with each tool you select. Here I’m using the Eye Refine Brush so it automatically shows me the eye masking.
Perfect Portrait gives you several workflow options as well. It is a
stand-alone program but can also open in Aperture, Lightroom, or
Photoshop. I like having this option and since I had the opportunity to
use both the entire suite and just Perfect Portrait, I can tell you that
the workflow is a little different in each.In the suite, you open the program and it goes to the Layers section by default. Then you open an image and use whichever program you’d like. If you opt to go with just Perfect Portrait without the suite, when you open the program you are first asked to open an image and then it opens the image in the program. No big deal, you say? Well, maybe not on the beginning part but each time you are done working on an image, after you save it, the program closes and you have to go through the process again! I found that to be both annoying and slower than just opening images in an already open program.
Let’s go through a sample workflow situation here on a single image using Adobe Lightroom. The program suggests you do any major color corrections and tonal adjustments first in your program of choice, a little strange because then the program will automatically make color adjustments. Maybe the emphasis here is on major. I found the easiest way was to import the file in Lightroom, do any quick adjustments there, then go to Perfect Portrait from the File/Plugin Extras menu. The program will automatically go to work on your image and if you like what you see, you’re done! If you think it should be fine-tuned, choose one of the many presets included under the proper category. For example, if you’re retouching a man’s portrait, there are 12 presets included that range from Default, Elderly, Twilight, Warm, and more. The default menu shows up as a thumbnail bar across the bottom of the program and you can click on and apply whichever one you feel suits the image best. Once you’ve got the basics done, you can either quit there or use the tools to do more work.
If the default enhancements aren’t to your
liking, you can choose from several preset looks that come with the
program or save your own. Hovering over each preset gives you a larger
sample and a short explanation. Model: Faith Dittmar.
Usually the first tool I’d grab would be the Retouch Brush, which is
similar to the Healing Brush in Photoshop. It works really fast, and can
be used to either dot out blemishes or drag over an area like bags
under the eyes. You can vary the opacity and also adjust brush size
using the bracket keys. I found it did a really great job of cleaning up
blemishes and keeping the skin realistic looking. There is also
pressure control for Wacom tablets. Then you can move to the Refine
Brush for the skin, eyes, and mouth if you feel the need to make
adjustments to those areas.One thing I found very useful was the ability to see just what the program was masking out using the Overlay view. Once again, if you are happy with the area the program selected, then leave it alone. If not, you can paint in and out using the Brush tool to have the exact area you want.
When you are done refining your image with the tools, then you advance to the slider menus on the right side of the interface. Also there you’ll find a Navigator and hot keys to select the face magnification, a Layer palette for each face, and sliders for fine-tuning skin color, shine, and other features.
I found the Face Size feature perhaps incorrectly named. Under that menu it lists Small, Medium, Large, and Extra Large, like you’re buying a man’s shirt. When I first saw it, I thought maybe it changed the shape of the face. Not so, as already noted. What it does is define how tightly or loosely you’d like the automatic face detection feature to work. Small will crop in really closely while Extra Large will stray further into the neck, hair, and other features. While I think it’s a good idea, especially if you’re trying to batch process images and don’t want to look at everyone, maybe either a number system or words like Tight or Loose would make more sense.
Several split-screen viewing options are
available so you can see not just the retouching but changes in color
and contrast to the image. Model: Kate Consavage.
Once you have fine-tuned an image and gotten it just the way you
like, you may not want to go through all that again on similar images.
Or you may just want to save your work as a “look” for your brand. No
fear. You can save and label all the work you’ve just done as a custom
preset to be called up whenever you’d like.Let’s take a look now at how Perfect Portrait handles multiple faces in an image. When you open an image with multiple faces, the software automatically detects each face and places it on its own layer. If for some reason it missed a face, usually because it was not facing the camera, you can add the face manually. I did try to see how it would do with an image with 11 faces in a full-length pose. No faces were selected, so there are limits! But I then pulled up an image with three faces and it worked just fine. You can work on each face on its own layer independently.
You also have options when saving the image. You can just hit the Save button and your file will be saved as a PSD layer, your original file on the bottom layer and the retouched file on top. This is in keeping with nondestructive editing so your untouched original can always be accessed. You also have the option of using Export from the File menu to save as a TIFF or flattened JPEG file.
When a file is selected with more than one
face, Perfect Portrait detects each face and places it on its own layer
so you can make adjustments to each face individually. If a face was
missed because it was too small or not facing the camera, you can
manually add it. Models: The Tromba Family.
Now let’s talk about batch processing and how it can affect your
workflow. Many photographers like to “pretouch” their images, or do
modest retouching of each image from a session before they show them to a
client. Since most days I barely have enough time to eat my bowl of
Frosted Mini-Wheats in the morning, I’m not a big fan of retouching 50
images when the client may only buy two or three of them. Seems like a
significant amount of time spent with little reward. That may change
now.You see, I can open Lightroom, import a folder of images, export them to Perfect Portrait, choose the options I’d like, and press Export once again. VoilĂ ! All images are pretouched. I put four JPEGs in a folder to test and it went through them flawlessly. It did take a little while even with JPEGs so if you have lots of images to do you may want to hit the Export button and then go have your Frosted Mini-Wheats! And speaking of time, I have a rather new computer I used to test this. It has 4GB of RAM and a quad processor so take the minimum specs required pretty seriously, as this program does take some processing power.
Like most current software companies, onOne has extensive support online. They have a Knowledge Base that allows you to search for answers to common questions and e-mail support. They also have a feature I found most useful, onOne University, which has many tutorial videos. Because of the newness of the program, there are only a few on Perfect Portrait, but they do a good job of explaining the basics and will get you up and running quickly.
When faces are too small for the program
to detect, a bounding box pops up that allows you to select them
yourself. In cases like this image with 11 people, it’s probably faster
and more accurate to manually retouch.
I believe onOne has created an application that can be of great
benefit to both amateurs and professionals. Amateurs will appreciate the
short learning curve and being able to quickly improve their images.
Professionals will be able to integrate Perfect Portrait into their
workflow for several applications, from pretouching to fully finished
files, using it either as a stand-alone product or with their usual
product of choice like Aperture, Lightroom, or Photoshop. In particular,
the ability to batch process files without having to stop for each
image is of great value.Note: It is recommended that you use an Intel Core 2 Duo, Xeon, or better processor. In addition, 8GB of RAM is recommended, while 4GB of RAM is the minimum you should use.
Perfect Portrait sells for $99.95, with an upgrade price of $69.95. Perfect Photo Suite 6 sells for $299.95, with an upgrade price of $149.95. For more information, visit onOne Software’s website at: www.ononesoftware.com.
Subscribe to:
Posts (Atom)